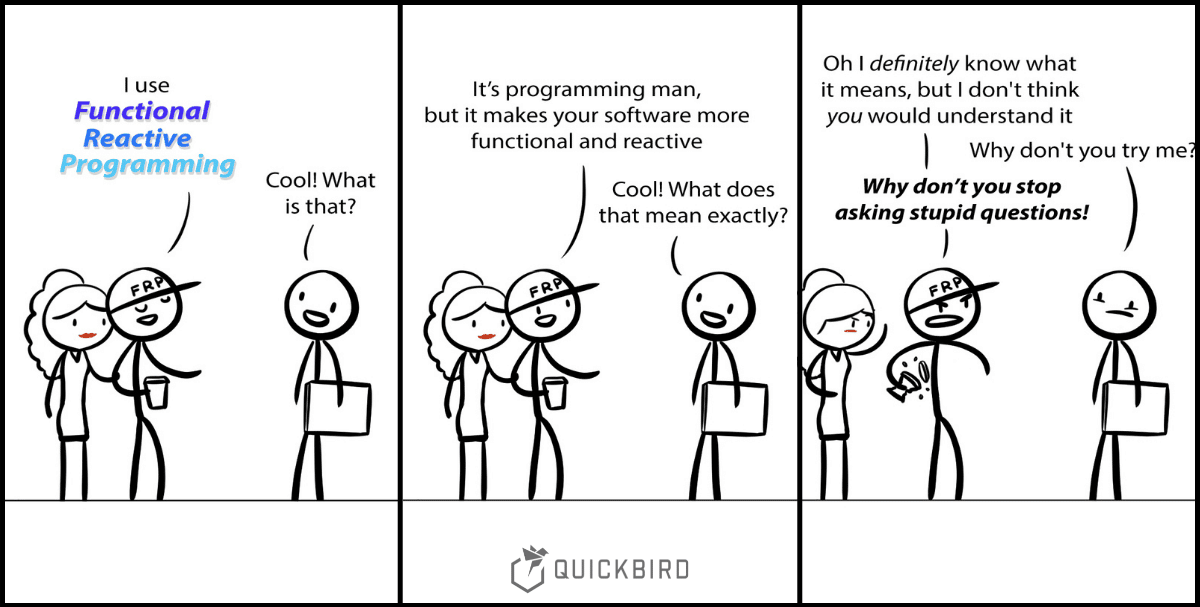
What is Functional Reactive Programming (FRP)?
The new buzzword to impress your friends: Functional Reactive Programming (FRP)
Using Functional Reactive Programming, you write less code, you have fewer bugs, you can program asynchronously without killing yourself, it’s just awesome. That’s what they told me. Everybody talks about it but many people don’t really know what it fundamentally is. So what is it, at its very core? I mean, without the buzzwords, without the cryptic Rx/Observable/Subscriber keyword bullshit.
High-level view
To understand what Functional Reactive Programming is, first, you have to split this long clunky word apart:
Functional Reactive Programming = Functional Programming + Reactive Programming
That simplifies the problem at least a bit. Functional Reactive Programming is a programming paradigm that is based on functional programming and reactive programming. So we have to understand these two first to understand what’s the whole thing.
What is functional programming?
Here is the definition of Wikipedia on Functional Programming:
“functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data”
That means in essence that in functional programming everything is expressed as a mathematical function.
Not really. Mathematical functions like the following have a property that many normal Java functions do not possess:
f(x) = y
They always give you the same output value (y) for a given input value (x).
g(a,b) = a + b
If I put in a=1, b=1 I will always get 2 as a result, no matter how often I calculate/execute that. In non-functional programming environments that is not always the case:
The result of the function will be different when I call it twice vs. only once. It changes a state that is outside of the function. That makes the result unpredictable. My program will behave differently depending on the number of executions.
That’s why functional programming is especially popular in the area of Research and Math. It allows you to prove mathematically that your program works as expected. Your function does not have any (mutable) state. It’s 100% predictable what the output of a certain input will be. Functional programming languages like Haskell even enforce this. Everything is a function and there is no state.
In non-functional programming languages (probably everything that you work with) there is no guarantee what will happen. You have the ability to save state. This is useful and necessary but it also makes your software less predictable. For example, bugs might arise after a server ran for 2 days, or after you pressed that button in an app too often.
That’s why many non-functional programming languages include elements from functional programming e.g. in their standard library. They offer functions like filter/map/reduce which make sub-parts of your software behave in a functional way. So in the end, not your whole software is functional but many critical sub-parts. This helps to prevent bugs.
What is Reactive Programming?
Reactive Programming is a paradigm that deals with data flow.
Reactive libraries or programming languages…
- …allow you to easily express data flows.
- …automatically evaluate your data flows and propagate data changes.
It becomes clearer when we look at an example:
You can see that the key difference in reactive programming is that state changes propagate automatically according to our defined data flow (a := b + c). In imperative programming, you always have to actively trigger those state changes.
So what is Functional Reactive Programming?
As mentioned, it’s the combination of the functional programming paradigm and the reactive programming paradigm. You might not be aware of it but you already know and did Functional Reactive Programming.
In fact, you did it pretty often… No way?
Take a look at Excel:
Excel offers functional and reactive programming paradigms to the user. These two paradigms are even a big reason why Excel is so popular. It allows you to calculate stuff reliably (functional – same input, same output) and it propagates those changes through your excel sheet (reactive – through defined data flows).
In practice
So how can I use Functional Reactive Programming? Most often you will use libraries for that like RxJava (or any other ReactiveX library). They allow you to model functional data flows in an easy manner.
These data flows are like tubes with certain connectors that do something with the tube’s content (data).
At the beginning always sits a data producer, the keyboard in that case. At the end of the data flow, represented by the tubes above, there is a subscriber/listener. The subscriber/listener wants to act on the result, for example, to update the UI of your software. In between, you have connectors, also called Operators: Here we have a filter connector and a map connector that manipulate data when it flows through the tube. That’s how you model all of your data flows if you use libraries like RxJava.
In code, the example above would like this with RxJava:
If this introduction got you excited, take a look at our blog article on how to write functional reactive User Interfaces. Or, if you first want to know more about the topic check out this great tutorial on FRP.
Conclusion
We love Functional Reactive Programming and use it in almost every app (via RxJava/RxKotlin/RxSwift).
The functional part of it helps to write more predictable software with fewer bugs.
The reactive part it allows you to write responsive UIs that update automatically when the corresponding data changes somewhere in your app.
It takes some time getting used to it since the concept is fundamentally different from what most people are used to. After an initial learning period though, it’s easy to fall in love with it ❤